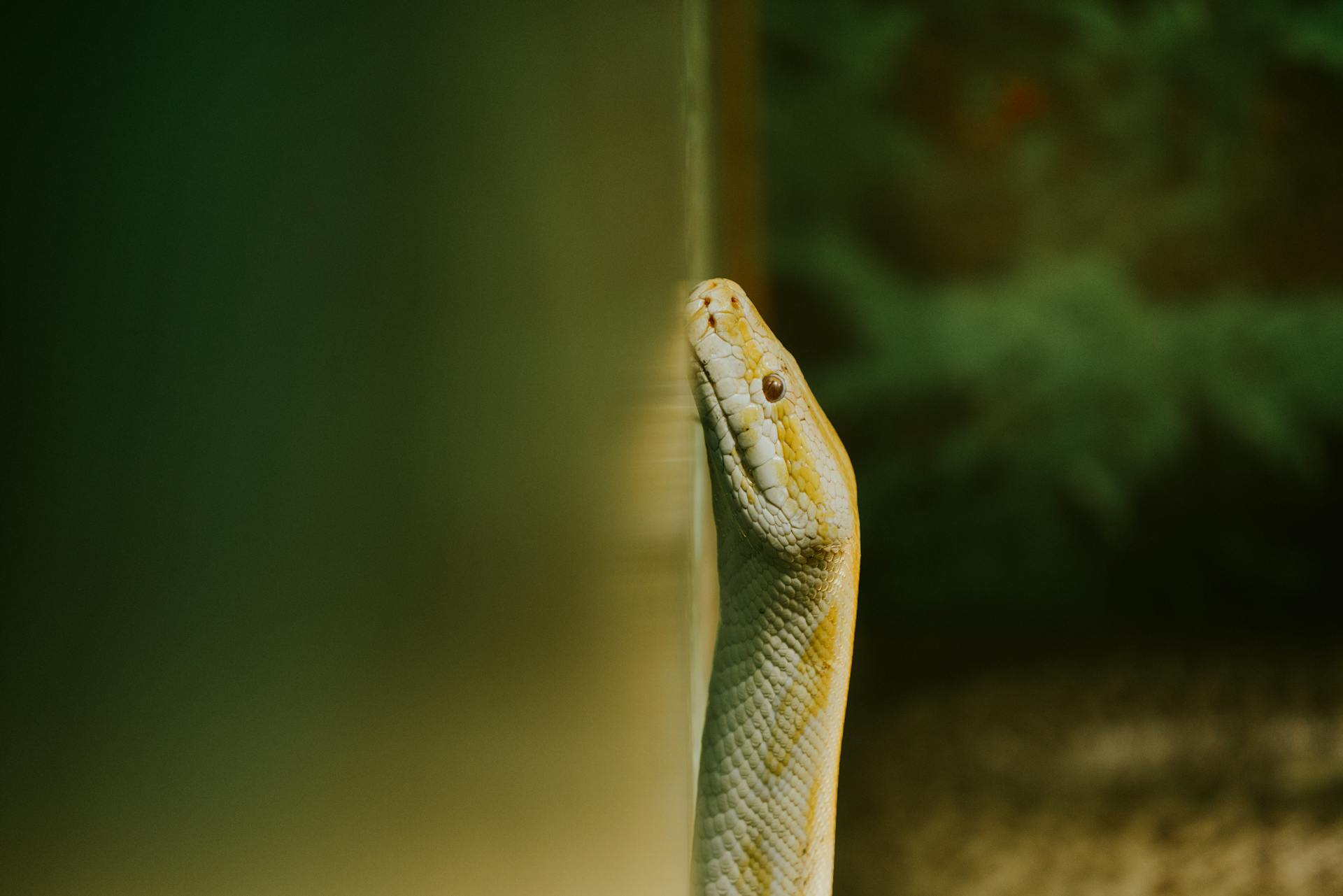
Cracking the coding interview in Python requires a deep understanding of the language and its applications. With the right preparation and strategies, you can confidently tackle even the toughest interview questions.
The key to acing a Python coding interview is to have a solid grasp of fundamental data structures and algorithms. You should be able to implement common data structures like linked lists, stacks, and queues, as well as sort and search algorithms like merge sort and binary search.
Practice is essential to solidifying your understanding of these concepts. Start by implementing basic data structures and algorithms from scratch, then gradually move on to more complex problems. By doing so, you'll develop a strong foundation and be better equipped to handle the challenges of a coding interview.
Check this out: Grokking Data Structures
Preparation
Preparation is key when it comes to acing a coding interview. To prepare effectively, you should focus on mastering common coding patterns, rather than trying to solve every possible problem on platforms like LeetCode.
LeetCode, which has over 3,000 problems, can be overwhelming, but the truth is that most problems can be broken down into just two dozen common coding patterns. By identifying these patterns, you can quickly solve problems and become more efficient in your preparation.
Here are the essential steps to take when preparing for a coding interview:
- Pick a good programming language to use
- Plan your time and tackle topics and questions in order of importance
- Combine studying and practicing for a single topic
- Accompany practice with coding interview cheat sheets to internalize the must-dos and must-remembers
- Prepare a good self introduction and final questions
- Try out mock coding interviews (with Google and Facebook engineers)
- (If you have extra time) Internalize key tech interview question patterns
Remember, the goal is to be strategic and efficient with your preparation, not to try to memorize every possible problem.
Why Is It Important?
Preparation is key to acing the coding interview. Mastering coding interviews is crucial for career advancement, as top tech companies like Google, Facebook, Amazon, Apple, and Netflix (often referred to as FAANG) use coding interviews as a primary method of evaluating candidates.
The skills you develop while preparing for coding interviews are directly applicable to real-world software development challenges. This means that the time you invest in preparation will pay off in the long run.
Grokking coding interviews enhances your ability to think algorithmically, which is essential for writing efficient and scalable code. This skill is highly valued by tech companies and can give you a competitive edge in the job market.
Being well-prepared for coding interviews can significantly boost your confidence, helping you perform better under pressure. This is especially important in high-stakes interviews where a little extra confidence can make all the difference.
Comprehensive Prep in 99
The key to comprehensive coding interview prep isn't to drill as many problems as possible. Rather, it's about being strategic and efficient with the problems you do study.
Educative-99 is a streamlined coding interview prep resource that whittles down the to-do list to just 99 hand-selected problems of varying difficulty levels. Each problem represents one of the 26 comprehensive patterns that can be used to solve almost any coding interview problem.
These 26 patterns can be used to solve the overwhelming majority of the 3,000 problems on LeetCode, which means that mastering them will give you a solid foundation for acing any coding interview.
By focusing on these essential coding interview patterns, you can avoid the redundancy and structureless approach that comes with trying to solve every potential problem on LeetCode.
Patterns and Techniques
Groking the coding interview in Python requires a deep understanding of common patterns and techniques.
Many coding interview questions follow certain patterns, and learning to recognize these patterns can significantly speed up your problem-solving process. Some common patterns include Two Pointers, Sliding Window, Fast and Slow Pointers, Merge Intervals, Cyclic Sort, and Tree Depth-First Search.
Developing a systematic approach to problem-solving is vital, which includes understanding the problem statement, identifying edge cases, developing a solution strategy, implementing the solution, and testing and debugging.
Some common coding interview patterns with examples include Reverse a String, Merge Similar Items, and Longest Continuous Subarray. These patterns can be practiced and learned to identify through resources like Educative-99, which includes 26 essential coding interview patterns.
To internalize key tech interview question patterns, you can use resources like AlgoMonster, which condenses software engineering coding interview questions into a set of key patterns. This can help you solve any long tail problem that is outside the set of commonly asked coding interview questions.
A different take: Mlops Interview Questions
Here are some common coding interview techniques that can be applied when you are given questions which you have never encountered before, and to get out of being stuck:
- Two Pointers
- Sliding Window
- Fast and Slow Pointers
- Merge Intervals
- Cyclic Sort
- Tree Depth-First Search
To maximize what you get out of your practice, you can refer to coding interview cheatsheets while you are studying and practicing. These can cover the best learning resources, must remembers (tips, corner cases) and must do practice questions for every data structure and algorithm.
Data Structures and Algorithms
Data Structures and Algorithms are the building blocks of coding interviews. A solid grasp of fundamental data structures is crucial, including Arrays and Strings, Linked Lists, Stacks and Queues, Trees and Graphs, Hash Tables, and Heaps.
To truly understand data structures, try implementing them from scratch, which will give you a deeper understanding of how they work internally and when to use them effectively. Understanding when and how to use these data structures efficiently is key to solving many coding problems.
Some common data structures and algorithms include:
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees and Graphs
- Hash Tables
- Heaps
- Sorting and Searching
- Recursion and Dynamic Programming
- Greedy Algorithms
- Divide and Conquer
- Graph Algorithms
Implementing Data Structures
Implementing Data Structures from Scratch is a great way to truly understand how they work internally. This exercise will give you a deeper understanding of how they work and when to use them effectively.
Understanding data structures from scratch requires a solid grasp of fundamental data structures. These include arrays and strings, linked lists, stacks and queues, trees and graphs, hash tables, and heaps.
Implementing data structures from scratch can be a challenging but rewarding experience. It's a great way to learn by doing and to develop problem-solving skills.
Here are some key data structures to focus on when implementing from scratch:
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees and Graphs
- Hash Tables
- Heaps
By implementing data structures from scratch, you'll be able to identify common pitfalls and areas for improvement, making you a better programmer in the long run.
Time and Space Complexity
Time and Space Complexity is a crucial aspect of Data Structures and Algorithms. Understanding Big O notation is essential to analyze the efficiency of your solutions.
Being able to analyze the efficiency of your solutions in terms of time and space complexity is crucial, as mentioned in Example 5. This involves understanding Big O notation and being able to optimize your code for better performance.
Analyzing time and space complexity will help you develop the ability to quickly evaluate different solutions during an interview, as mentioned in Example 1. This means you'll be able to identify the most efficient solution among multiple options.
Providing a working solution without considering its efficiency is a common pitfall, as highlighted in Example 6. Always analyze and discuss the time and space complexity of your solution, and be prepared to optimize if needed.
Some common patterns that can help you analyze time and space complexity include:
- Two Pointers
- Sliding Window
- Fast and Slow Pointers
- Merge Intervals
- Cyclic Sort
- In-place Reversal of a LinkedList
- Tree Breadth-First Search
- Tree Depth-First Search
- Two Heaps
- Subsets
- Modified Binary Search
- Top K Elements
- K-way Merge
- Topological Sort
These patterns can help you recognize common problems and develop efficient solutions, but remember to always analyze time and space complexity to ensure your solution is the best it can be.
Algorithms
Algorithms are a crucial part of data structures and computer science in general. Familiarity with common algorithms and their applications is essential to solving many coding problems. Some important categories include Sorting and Searching, Recursion and Dynamic Programming, Greedy Algorithms, Divide and Conquer, and Graph Algorithms.
Sorting and Searching algorithms are used to arrange data in a specific order or find specific data within a dataset. Recursion and Dynamic Programming are used to solve problems by breaking them down into smaller subproblems. Greedy Algorithms make the locally optimal choice at each step, which often leads to a global optimum solution. Divide and Conquer algorithms divide a problem into smaller subproblems, solve each subproblem, and then combine the solutions to solve the original problem.
Here are some key Graph Algorithms to know:
- Breadth-First Search (BFS)
- Depth-First Search (DFS)
- Dijkstra’s Algorithm
- Bellman-Ford Algorithm
- Floyd-Warshall Algorithm
- Topological Sort
Understanding when and how to use these algorithms efficiently is key to solving many coding problems. By mastering these algorithms, you'll be able to tackle a wide range of coding challenges and impress potential employers with your problem-solving skills.
Consider reading: Grokking Algorithms
Key Components
To truly grok coding interviews, you need to understand their key components.
Data structures and algorithms are the foundation of coding interviews. They're the building blocks of problem-solving, and understanding them is crucial to acing an interview.
Big O notation is a key concept in coding interviews, as it helps you analyze the time and space complexity of algorithms.
Arrays and linked lists are fundamental data structures that are often used in coding interviews. They're simple yet powerful, and understanding how to use them is essential.
Greedy algorithms are a type of algorithm that make the locally optimal choice at each step, with the hope of finding a global optimum solution. They're often used in coding interviews to solve problems related to optimization.
Suggestion: Grokking Algorithms Second Edition
Evaluation Criteria
In a coding interview, you'll be evaluated on four key criteria. These are the things that top tech companies always look for, and they'll help you determine whether you're a good fit for the job.
Communication is key, and you'll be expected to ask clarifying questions and explain your approach clearly. This means you should be able to articulate your thought process and tradeoffs without leaving the interviewer in the dark.
Problem solving is another crucial aspect, and you'll be tested on your ability to understand the problem, approach it systematically, and discuss multiple potential solutions. You should also be able to accurately determine time and space complexity and optimize them.
Technical competency is also important, and you'll be expected to translate your solutions into working code without too much struggle. This means you should have a strong knowledge of language constructs and be able to write clean, correct code.
Testing is the final criterion, and you'll be expected to test your code against both normal and corner cases. This means you should be able to identify and self-correct any issues in your code.
Here are the four evaluation criteria in a concise list:
- Communication: Asking clarifying questions and explaining your approach clearly.
- Problem solving: Understanding the problem, approaching it systematically, and discussing multiple potential solutions.
- Technical competency: Translating solutions into working code with no significant struggle.
- Testing: Testing code against normal and corner cases and self-correcting issues.
AlgoMonster
AlgoMonster is an excellent platform created by Google engineers that uses a data-driven approach to condense software engineering coding interview questions into a set of key patterns.
It's a structured, easy-to-digest course that condenses coding interview questions into key patterns, making it a great resource for learning and practicing.
AlgoMonster is similar to LeetCode, but with only the key patterns you need to know, allowing you to focus on mastering the most important concepts.
The platform covers data structures and algorithms questions in all the common languages, including Python, Java, C#, JavaScript, C++, Golang, and more.
By using AlgoMonster, you can master important coding interview data structures and algorithm questions through practice and easy-to-understand guides.
You can even join AlgoMonster today for a 70% discount, making it an affordable resource for learning and practicing.
Here are some of the key features of AlgoMonster:
- Data-driven approach to condense coding interview questions into key patterns
- Structured, easy-to-digest course
- Covers data structures and algorithms questions in all common languages
- Master important coding interview data structures and algorithm questions through practice and guides
- Affordable with a 70% discount available
Tree BFS
Tree BFS is a fundamental concept in data structures and algorithms. It involves traversing a tree level by level, starting from the root node.
The pattern of Tree BFS involves several problems, including Binary Tree Level Order Traversal, Reverse Level Order Traversal, and Zigzag Traversal. These problems are all considered easy to medium in difficulty.
One of the key applications of Tree BFS is finding the level averages in a binary tree, which is a simple yet useful problem. The level averages problem is a great example of how Tree BFS can be used to solve real-world problems.
To visualize the pattern of Tree BFS, consider the following list of problems:
- Binary Tree Level Order Traversal (easy)
- Reverse Level Order Traversal (easy)
- Zigzag Traversal (medium)
- Level Averages in a Binary Tree (easy)
- Minimum Depth of a Binary Tree (easy)
- Maximum Depth of a Binary Tree (easy)
- Level Order Successor (easy)
- Connect Level Order Siblings (medium)
- Problem Challenge 1: Connect All Level Order Siblings (medium)
- Problem Challenge 2: Right View of a Binary Tree (easy)
These problems demonstrate the versatility and importance of Tree BFS in data structures and algorithms. By understanding and mastering Tree BFS, you can tackle a wide range of problems and improve your skills as a programmer.
Bit Manipulation
Bit manipulation is a powerful technique for solving certain problems efficiently. It involves performing operations on individual bits within a binary number.
The key operations in bit manipulation include AND, OR, and XOR, which are used to combine bits in different ways. These operations can be used to set, clear, or toggle individual bits.
Bit shifts, specifically left and right shifts, are also essential in bit manipulation. They allow you to move the bits of a number to a different position, effectively multiplying or dividing the number by a power of 2.
Here are some common bit manipulation operations and their effects:
Bit manipulation is particularly useful in problems that involve counting or manipulating individual bits within a binary number, such as counting the number of set bits in an integer.
Frequently Asked Questions
How long does it take to finish grokking the coding interview?
Estimated completion time: 2-4 weeks for experienced programmers studying intensively 3-4 hours a day
Is Python ok for coding interviews?
Yes, Python is a great choice for coding interviews, thanks to its powerful and convenient standard data structures that save you time and let you focus on problem-solving. With Python, you can focus on the logic and creativity of the problem, rather than getting bogged down in data structure implementation details.
How many problems are in Grokking the Coding Interview?
Grokking the Coding Interview contains 99 essential coding interview challenges, carefully curated with hiring managers from top tech companies. Master these challenges and boost your chances of acing your next coding interview.
Sources
- https://www.educative.io/courses/grokking-coding-interview
- https://github.com/dipjul/Grokking-the-Coding-Interview-Patterns-for-Coding-Questions
- https://grokkingtechinterview.com/grokking-coding-interviews-with-99-essential-problems-7838ae2a9ff6
- https://algocademy.com/blog/grokking-the-coding-interview-a-comprehensive-guide-to-mastering-technical-interviews/
- https://www.techinterviewhandbook.org/coding-interview-prep/
Featured Images: pexels.com