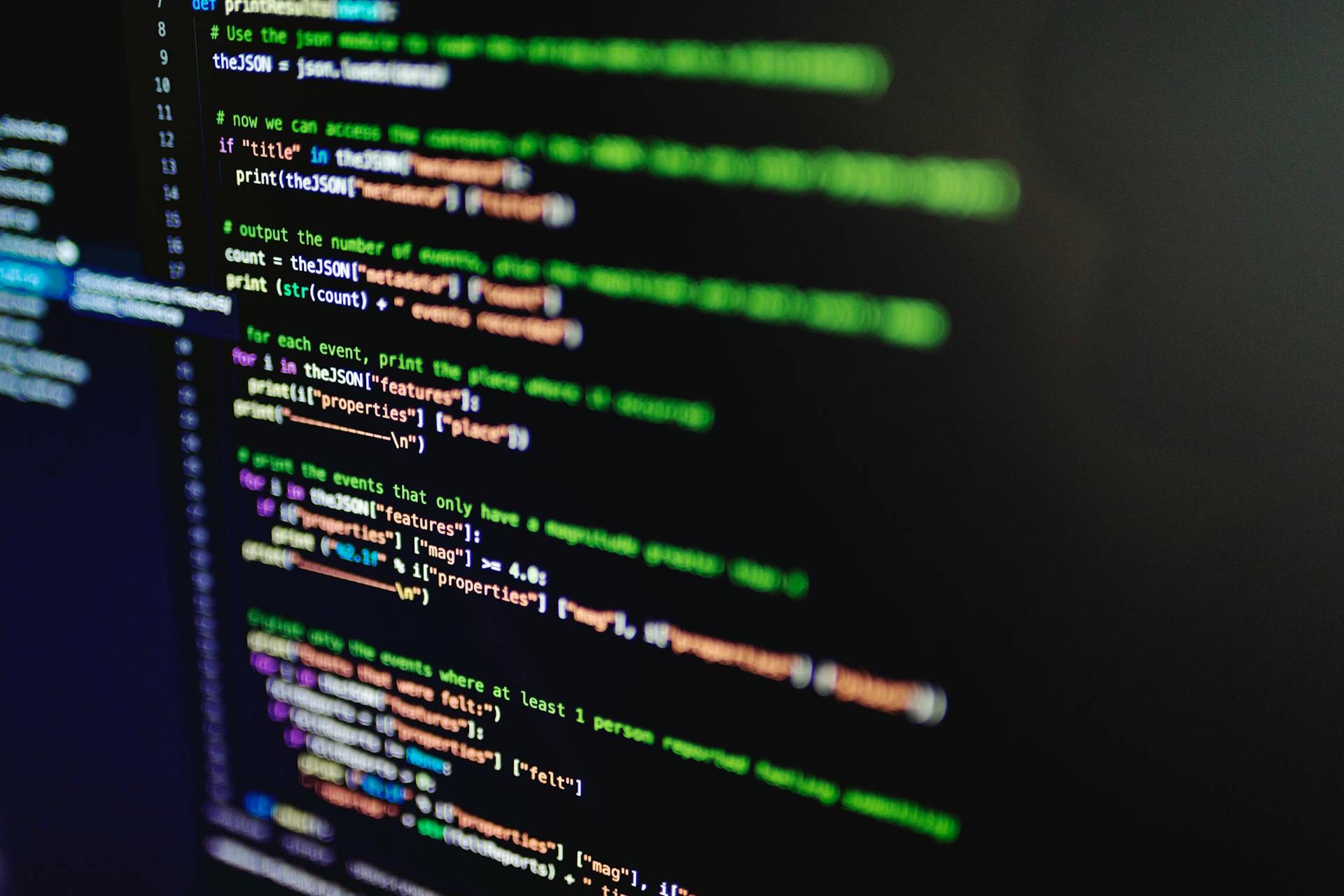
Learning assembly code is a great way to understand how modern computer systems work. It's a low-level programming language that allows you to write instructions that the computer's processor can execute directly.
Assembly code is specific to a particular computer architecture, such as x86 or ARM, and is used to write operating systems, device drivers, and other low-level software.
To get started with assembly code, you'll need to choose a specific architecture to focus on, such as x86 or ARM, and learn the corresponding instruction set. This will allow you to write assembly code that can be executed by the computer's processor.
Assembly code is typically written in a text editor and assembled into machine code using an assembler, such as nasm or gas.
A fresh viewpoint: Learning Systems in Machine Learning
What is Assembly Code?
Assembly code is a low-level programming language that uses symbolic representations to represent machine-specific instructions. It's the most basic form of code that computers understand.
Each instruction in assembly code is unique to a specific computer architecture, making it difficult to write portable code. This is in contrast to high-level languages like C or Python, which can run on multiple platforms with minimal modifications.
Assembly code is typically used for tasks that require direct hardware manipulation, such as optimizing performance-critical code or working with low-level system resources. This can include tasks like device drivers, firmware, and operating system development.
Assembly code is made up of mnemonics, which are symbolic representations of machine-specific instructions. These mnemonics are often represented by a combination of letters and numbers, making it easier to write and read code.
Relevance Today
Learning assembly language programming is still relevant today because it helps optimize the speed of the computer and creates a program that runs quicker than high-level language programs can.
While many programmers prefer high-level languages, there are still many benefits to understanding assembly, especially for traditional programs and complex applications.
Broaden your view: Best Way to Learn to Code Veteran Programs
Assembly language is useful in reverse engineering and malware analysis, which can be a valuable skill in certain fields.
Understanding assembly language can give you a deeper understanding of how computers work, which can be a valuable asset in your programming career.
Learning assembly language can also help you debug more effectively, as it allows you to tell the computer not just what to do, but how to do it.
There are still many benefits to learning assembly language, even in today's world of cloud-based applications.
A fresh viewpoint: What Is the Hardest Code Language to Learn
Getting Started
You can start assembly language programming by taking an online course like Cybrary, which provides interactive modules to help master the language. This will give you the skills to write and read assembly programs, understand different data representations, and more.
To execute assembly language, you'll need to follow these steps: write assembly code, assemble the code, generate an object file, link and create executables, and finally run the program.
To get started, you can use a text editor to write assembly code, save it with a proper extension like .asm, .s, or .asmx, and then use an assembler to convert it to machine language.
Curious to learn more? Check out: Learn How to Code Google's Go Programming Language
How to Execute?
To execute assembly language, you'll need to write the code first. Open any text editor on your device and write the mnemonic codes, saving the file with a proper extension like .asm, .s, or .asmx.
You'll then need to assemble the code, which involves converting it to machine language using an assembler. This will generate an object file with an extension of .obj.
To make your assembly language executable, you'll need to link it to libraries. You can use a linker like lk for this purpose.
Here's a step-by-step summary of the process:
- Write assembly code
- Assemble the code
- Generate an object file
- Link the code to libraries
- Run the program
Starting Programming
Starting Programming can be intimidating, but it's easier than you think. You can start by taking an online course like Cybrary's assembly language course, which allows you to learn at your own pace.
Cybrary's course covers the basics of assembly language programming, including writing and reading assembly programs, understanding different data representations like Binary, hex, and 2's compliment.
You can also learn assembly language through books or online blogs, but taking an online course like Cybrary provides interactive modules to help master the language.
With an assembly certification, you'll gain the ability to address critical computer performance issues and give the processor specific instructions.
Consider reading: Learn Morse Code Online
One Answer
You can start assembly language programming by taking an online course like Cybrary, which provides interactive modules to help master the language.
To write assembly code, simply open any text editor on your device and write the mnemonic codes in it, saving the file with a proper extension like .asm or .s.
Assembling the code converts your code to machine language using an assembler, generating an object file with an extension of .obj.
Linking and creating executables involves linking multiple source codes to libraries to make them executable, using a linker like lk for this purpose.
To run the program, create an executable file and then run it as usual, depending on the software being used.
The compilation process involves converting high-level code into machine code, which can be understood by the computer's hardware.
Writing your own assembly code allows you to see how closely assembly code maps to binary code.
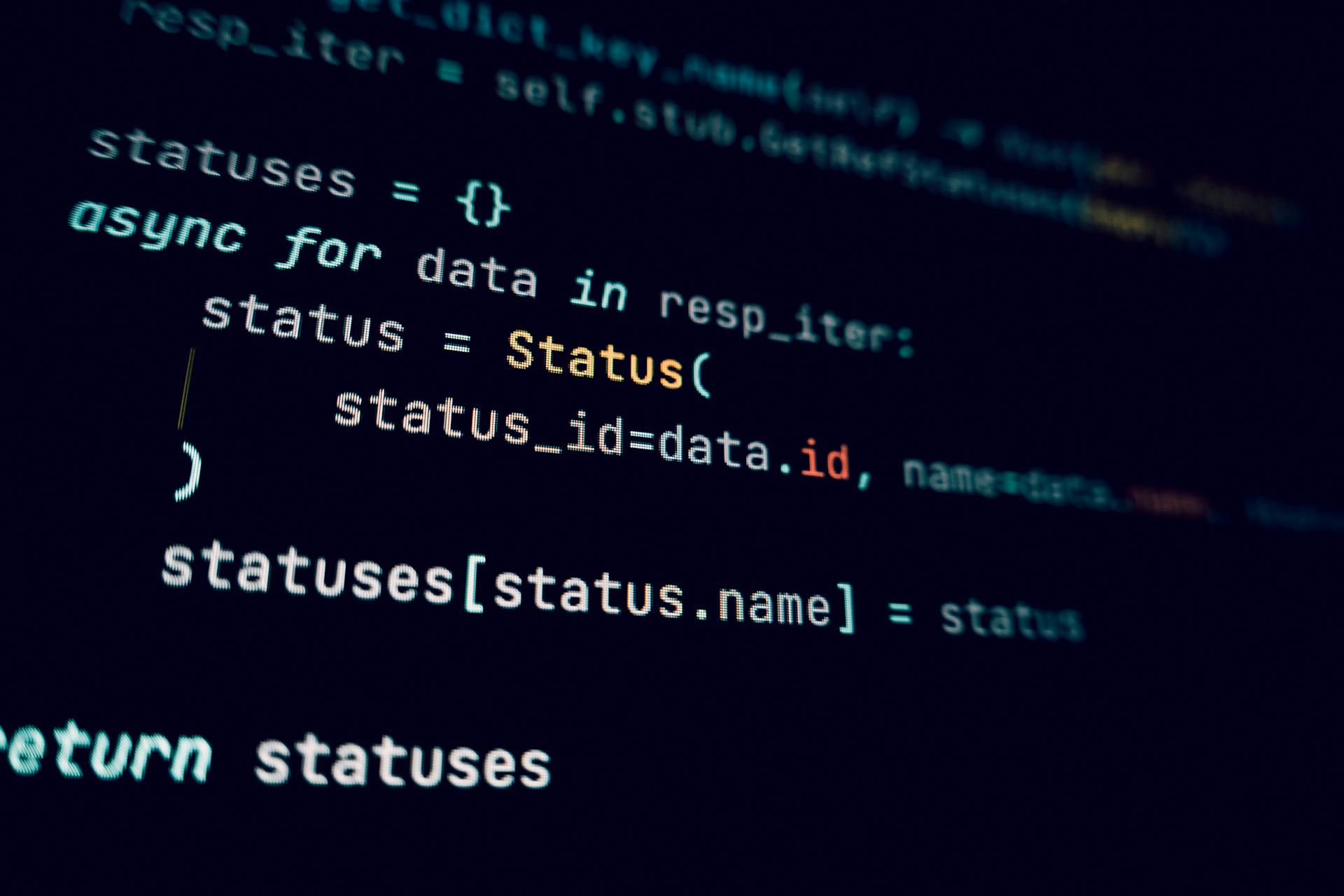
Students can earn an assembly certification, increasing their ability to address critical computer performance issues and give the processor specific instructions.
Here's a step-by-step guide to executing assembly language:
- Write assembly code in a text editor.
- Assemble the code using an assembler.
- Generate an object file with an extension of .obj.
- Link the code to libraries using a linker like lk.
- Create an executable file.
- Run the program as usual.
Tools and Concepts
To learn assembly code, you'll need to understand the basics of machine language. Machine language is the only language that a computer can understand directly.
Assembly code is a low-level programming language that uses symbolic representations of machine code instructions. It's translated into machine code by an assembler.
A key concept in assembly code is the use of registers, which are small amounts of memory inside the CPU that hold data temporarily.
Computer Architecture Course Reviews
Computer Architecture Course Reviews are a great way to gauge the quality of a course, and in this case, the ratings speak for themselves. 45% of students gave the Assembly Language course a perfect 5 stars.
The majority of students, 45%, were extremely satisfied with the course, awarding it 5 stars. This suggests that the course is well-structured and effectively taught.
A smaller but still significant group, 24%, gave the course 4 stars, indicating a good but not exceptional experience. This could be due to various factors, such as the pace of the course or the instructor's teaching style.
20% of students gave the course 3 stars, which is a decent rating but indicates some areas for improvement. This could be due to issues with the course materials or the instructor's delivery.
A small minority, 5%, gave the course 2 stars, suggesting some significant problems with the course. This could be due to issues with the course's content or the instructor's teaching methods.
Only 6% of students gave the course a dismal 1 star, indicating a very poor experience. This could be due to a range of issues, from the course being overly difficult to the instructor being unhelpful.
Components of
Components of Assembly Language are the building blocks that help us create code. Registers are the fast memory locations inside the processor that help the Arithmetic Logic Unit (ALU) perform arithmetic operations and temporarily store data.
For more insights, see: Books to Help Learn Code in Java
Registers are like the processor's memory, and they're essential for storing data. The Accumulator (Ax), Bx, and Cx are examples of registers.
Commands in assembly code are instructions that tell the assembler what to do. They use self-descriptive abbreviations, like "ADD" for addition and "MOV" for data movement.
Commands are the backbone of assembly language, and they're what make the code work. An example of a command is "ADD", which stands for addition.
Instructions are the mnemonic codes we give to the processor to perform specific tasks. Examples include "LOAD", "ADDITION", and "MOVE".
Instructions are the heart of assembly language, and they're what make the code do something. The "ADD" instruction is a good example.
Labels are symbolic names or identifiers that indicate a particular location or address in the assembly code. They help us navigate the code and make it more readable.
Labels are like bookmarks in a book, and they help us find specific parts of the code. An example of a label is "FIRST", which indicates the starting point of the execution part of the code.
Mnemonics are acronyms for assembly language instructions or machine functions. They're like shortcuts that make the code easier to read and write.
Mnemonics are a key part of assembly language, and they're what make the code more readable. Examples of mnemonics include "ADD", "CMP", "MUL", and "LEA".
Macros are program codes that can be used anywhere in the program through a single call. They're often embedded with assemblers and compilers.
Macros are like reusable code snippets, and they save us time and effort. An example of a macro is "%macro ADD_TWO_NUMBERS 2".
Operands are the data or values we give to the instruction to perform an operation on. They're like the inputs and outputs of a mathematical equation.
Operands are an essential part of assembly language, and they're what make the code work. An example of operands is "R1" and "R2" in the instruction "ADD R1, R2".
Opcode is the mnemonic code that specifies the operation to be performed. It's like a recipe for the processor to follow.
Opcode is a key part of assembly language, and it's what makes the code execute correctly. An example of an opcode is "ADD", which stands for addition.
Here's a summary of the components of assembly language:
Assembler
An assembler is a program that converts symbolic assembly language into machine code that a CPU can execute. This process is crucial for creating executable files that can run on a computer.
There is no single standard for x86-64 assembly language, so it's essential to choose the right assembler for your needs. In this case, we're using Flat Assembler (FASM), which is a popular choice due to its simplicity and features.
FASM is a great option because it's small, easy to obtain and use, and has a nice macro system. It also comes with a handy little editor, making it a great choice for beginners and experienced programmers alike.
Here are some key features of FASM:
- Small and easy to use
- Has a nice macro system
- Comes with a handy little editor
Overall, an assembler is a vital tool for any programmer working with assembly language, and FASM is a great choice for those looking for a reliable and user-friendly option.
Registers
Registers are the fast memory locations situated inside the processor, helping the Arithmetic Logic Unit (ALU) to perform arithmetic operations and temporary storing of data. They're like a special kind of memory built right into the CPU.
There are many different kinds of registers, but we'll focus on the general-purpose registers, of which there are sixteen. Each of them is 64 bits wide, and for each of them the lower byte, word, and double-word can be addressed individually.
Here's a breakdown of the general-purpose registers:
The higher 8 bits of rax, rbx, rcx, and rdx can also be referred to as ah, bh, ch, and dh.
Memory and Addresses
Memory is a crucial part of computing, allowing data to be stored and retrieved efficiently.
A byte is the basic unit of memory, consisting of 8 binary digits or bits. In computer terminology, a byte is often used to refer to a single character.
Memory addresses are used to identify specific locations in memory where data is stored. Think of it like a house address, where each address corresponds to a specific location.
A 16-bit address can store 64KB of data, while a 32-bit address can store 4GB of data. This is why larger addresses can store more data.
In a 32-bit system, the address bus width is 32 bits, allowing for more memory to be addressed.
PE Format and DLL Imports
PE format is used for Windows executable files, which have a header that contains important information like the file's machine type and number of sections.
The PE file format is made up of several sections, including the .text section, which contains the program's code, and the .data section, which contains initialized variables.
The .idata section contains import tables, which list the DLLs a program needs to run.
DLL imports are used to link to external libraries, allowing a program to use functions and variables from other libraries.
The import table contains a list of DLLs that a program needs to run, along with the functions and variables it needs to access.
A program can use the LoadLibrary function to load a DLL at runtime, but this is not as efficient as using the import table.
The import table can be used to resolve external references to functions and variables, making the program more efficient and easier to debug.
64-Bit Windows Calling Convention
The 64-bit Windows calling convention is a crucial aspect of programming, especially when dealing with 64-bit systems. It uses the __stdcall calling convention, which is the default for 64-bit Windows.
This convention specifies that the last four bytes of the function's argument list are pushed onto the stack, followed by the return address. The function then executes, and when it returns, it pops the arguments off the stack.
The __stdcall convention is used for both 64-bit and 32-bit Windows, but the stack frame layout is different between the two. In 64-bit Windows, the function's return address is not stored on the stack.
The calling convention also specifies that the function's arguments are passed on the stack, with the first argument being the most recent addition. This is in contrast to the __cdecl convention, which requires the caller to clean up the stack.
In 64-bit Windows, the function's parameters are passed on the stack, but the caller is not responsible for cleaning up the stack. This is because the __stdcall convention takes care of popping the arguments off the stack when the function returns.
Frequently Asked Questions
How hard is it to learn assembly code?
Assembly code is considered one of the most challenging coding tools to learn and master due to its low-level, machine-specific nature. Learning assembly code requires dedication and a strong foundation in computer architecture and programming concepts.
Does anyone still write assembly code?
Yes, assembly code is still used today, but only in small amounts within larger systems for performance or hardware interaction reasons. It's a niche use case, but one that's essential for certain applications.
Sources
- https://www.geeksforgeeks.org/what-is-assembly-language/
- https://www.cybrary.it/course/assembly
- https://gpfault.net/posts/asm-tut-0.txt.html
- https://stackoverflow.com/questions/70098588/how-to-start-learning-assembly-language-on-any-system
- https://www.codecademy.com/learn/computer-architecture-assembly-language
Featured Images: pexels.com