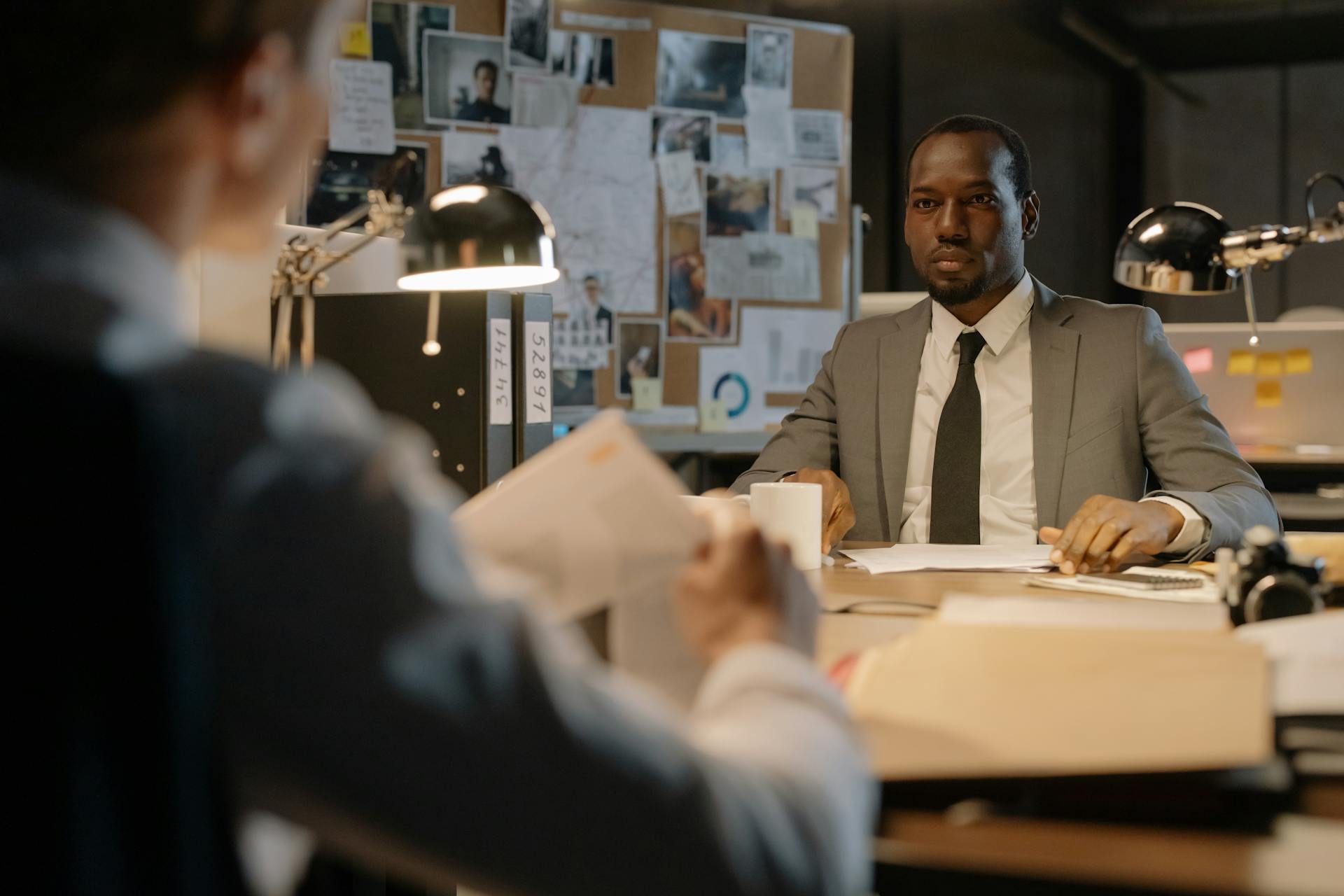
Outlier detection is a crucial step in data analysis, and Python is an ideal language for it. Pyod is a popular Python library that offers a range of outlier detection algorithms.
With Pyod, you can use the Auto-Sklearn algorithm to detect outliers in your data. This algorithm can handle a variety of data types and is particularly effective for high-dimensional data.
Pyod also provides the Local Outlier Factor (LOF) algorithm, which is useful for detecting outliers in data with a complex structure.
A unique perspective: Data Labeling in Machine Learning with Python Pdf
Choosing the Right Method
Start with easy methods like z-scores and MAD before trying more complicated ones. These simple techniques are often effective for detecting outliers in most datasets.
If you're working with data that has a lot of different features, consider using Isolation Forest. This algorithm is particularly well-suited for complex data.
Local Outlier Factor is a good choice when your data forms groups, as it can identify outliers within these groups.
Autoencoders are best for spotting unusual patterns that are hidden in complex data, making them a great option when you need to detect outliers in high-dimensional data.
Explore further: Data Labeling in Machine Learning with Python
Statistical Methods
Statistical methods are a great way to detect outliers in your data. They use basic math to figure out which data points are way different from what's expected.
One common statistical method is the Mean Absolute Deviation (MAD), which looks at how far away each piece of data is from the middle value (median). If a data point is way off from this middle value, it might be something unusual.
The Z-score method is another statistical method that tells us how far a data point is from the average, measured in standard deviations. If a data point's z-score is more than 3, it's usually considered weird.
Here are some things to keep in mind when using statistical methods:
- They can be thrown off by very unusual data points
- They work best if data doesn't change much over time and is normally spread out
Using Box Plots
Using box plots is a great way to visualize outliers and quartiles in numerical data. A box plot shows the distribution of data through their quartiles, with the first quartile (Q1) being the middle number between the minimum and median, and the third quartile (Q3) being the middle number between the maximum and median.
Box plots are ideal for small and simple data sets with few columns, making them a useful tool for outlier detection.
The dots in the box plot correspond to extreme outlier values, which can be validated by filtering the data frame and using the counter method to count the number of outliers.
Here's a summary of how box plots work:
By using box plots, we can identify outliers and gain insights into the distribution of our data, which can be a valuable asset in many fields, such as quality control, financial fraud detection, and healthcare analytics.
Minimum Covariance Determinant and Elliptic Envelope
Minimum Covariance Determinant is a common Outlier Detection approach that only works if your data is Normally distributed. If your data is not Normally distributed, this algorithm won't work.
You should use simple statistical methods to detect outliers if your data is Normally distributed. Unfortunately, if your data is not Normally distributed, you won't be able to use this algorithm.
Broaden your view: How to Use Huggingface Models in Python
To use Minimum Covariance Determinant, you need to define the "shape" of the data based on its distribution. This means you should consider samples that stand far enough from the initial shape as outliers.
The algorithm works by defining a hypersphere (ellipsoid) in the space of your features that covers the normal data. Any samples that fall outside this shape should be considered an outlier.
Sklearn has two functions for this Outlier Detection technique: Elliptic Envelope and Minimum Covariance Determinant. Elliptic Envelope uses an empirical approach to estimate the covariance, while Minimum Covariance Determinant uses a robust approach.
My personal choice is the Elliptic Envelope, as it is an easy-to-use algorithm.
Time Series
Time Series is a unique beast when it comes to outlier detection.
Outliers in Time Series are divided into two groups: point and subsequence (pattern) outliers. Point outliers are single abnormal samples.
For detecting point outliers, you can use Unsupervised Outlier Detection algorithms, which tend to work well for such outliers. These algorithms will help you compare real observations with smoothed values to find samples that might be considered point outliers.
Exponential and convolutional smoothers are also effective tools for identifying point outliers, especially in seasonal data with no trend and random walks Time Series respectively.
Detecting pattern outliers is a much more challenging task, requiring both identifying the normal pattern and comparing the abnormal one to historical data.
Intriguing read: Outlier Detection in Time Series
Machine Learning Models
Machine learning methods learn what normal looks like from your data and then spot the data points that don't fit this pattern. This is a key concept in outlier detection.
Isolation Forest is one such method that finds outliers by splitting the data into smaller bits until it isolates the odd ones out. It's good at dealing with complicated patterns and very accurate in finding groups of strange data points.
Local Outlier Factor (LOF) is another method that finds odd data points by looking at how crowded an area is. If a data point is in a much less crowded area compared to its neighbors, it's likely an outlier.
Isolation Forest doesn't assume your data is spread out in a certain way, making it a flexible choice. However, it can take a lot of computer power to run and might fit too closely to the small details of your data.
Here are some key differences between Isolation Forest and LOF:
- Isolation Forest is good at dealing with complicated patterns
- LOF finds odd data points by looking at how crowded an area is
- Isolation Forest doesn't assume your data is spread out in a certain way
- Isolation Forest can take a lot of computer power to run
- LOF might fit too closely to the small details of your data
PyOD Library
The PyOD library is a comprehensive tool for detecting outlying objects in Python.
It has a unified API, making it easy to use and a universal toolkit.
PyOD provides well-written documentation with simple examples and tutorials across various algorithms.
The library is optimized and parallelized, making it work quite fast.
PyOD works with both Python 2 and 3.
Here are some of the key features of PyOD:
- Unified API
- Well-written documentation with examples
- Variety of Outlier Detection algorithms
- Optimized and parallelized
- Works with Python 2 and 3
What Is Pyod?
PyOD is a comprehensive and scalable Python library for detecting outlying objects.
It has more than 30 Outlier Detection algorithms implemented.
This library provides a complete and easy to navigate documentation full of valuable examples.
PyOD is regularly updated and is well recognized among the Data Science community.
PyOD Utilities
PyOD provides several valuable utility functions that make the exploring process easier. These functions are designed to simplify tasks and provide useful tools for data analysis.
The generate_data() function is one such utility that can be used for synthesized data generation. This function allows you to create artificial data for testing and experimentation purposes.
PyOD also offers the generate_data_clusters() function, which can be used to generate more complex data patterns with multiple clusters. This is particularly useful when working with datasets that have multiple features and relationships.
Other utility functions provided by PyOD include wpearsonr(), which calculates the weighted Pearson correlation of two samples. This function is useful for analyzing the relationship between two variables and understanding how they correlate with each other.
Here's a list of some of the utility functions provided by PyOD:
- generate_data() for synthesized data generation
- generate_data_clusters() for generating complex data patterns with multiple clusters
- wpearsonr() for calculating the weighted Pearson correlation of two samples
- and others
These utility functions are an essential part of the PyOD library and make it a powerful tool for data analysis and outlier detection.
Installing Pyod
Installing Pyod is a straightforward process. You can easily install Pyod via pip.
It's worth noting that Pyod doesn't install Deep Learning frameworks like Keras and TensorFlow for you, so you'll need to install those separately.
Implementation and Evaluation
Our model did a great job and found all the anomalies we added. This is a significant achievement, especially considering the fake anomalies were designed to test its accuracy.
In this evaluation, we checked if our model was able to identify the anomalies we intentionally introduced. It successfully pinpointed each and every one of them, demonstrating its effectiveness in outlier detection.
If this caught your attention, see: Grid Search in Python
Prerequisites for Data Reading
To start working with data, you'll need to import the Pandas library, which is used for reading in, transforming, and analyzing data. This is a crucial step in any data analysis project.
Pandas is a powerful tool that can help you read your data into a data frame, making it easier to work with and analyze. This is especially useful when you have a large dataset.
To read your data into a data frame, you can use Pandas to import your data. This will allow you to start working with your data and performing analysis.
Worth a look: Code Analysis That Detects Weakness in Application
Displaying the first few rows of data can give you a sense of what your data looks like and what information it contains. For example, you can use the .head() method to display the first five rows of data.
The data frame will contain information about the dimensions of the banknotes in millimeters, including columns like length, left, right, bottom, top, and diagonal.
Data Generation
To test the outlier detection model, a fictitious dataset was generated, drawing 200 points at random from one distribution and 5 points at random from a separate shifted distribution. This created a clear distinction between inliers and outliers, making it easier to evaluate the model's performance.
The dataset consisted of two samples, one with 200 points in blue and the other with 5 points in orange. This visual representation helps identify the outliers, but in real-world scenarios, the goal is to detect them without prior knowledge.
The pandas dataframe starting point after data generation had one column for numerical values and a second column for ground truth, which can be used for accuracy scoring.
Discover more: Random Shuffle Dataset Python Huggingface
Review Results
Reviewing the results of our outlier detection model is a crucial step in ensuring its accuracy and effectiveness. We can see that the outliers were picked up properly, but some of the tails of our standard distribution were also flagged as outliers.
To get a better understanding of our model's performance, we can calculate accuracy, precision, and recall. In this example, the model was 90% accurate, but some data points from the initial dataset were incorrectly flagged as outliers. This highlights the importance of fine-tuning our model to minimize false positives and negatives.
To reduce the chances of wrongly labeling data as normal or weird, we can use various techniques such as cross-validation, graphing our model's decisions, combining models, and using the F1 score to find a good balance between precision and recall.
Here are some specific metrics to keep in mind when evaluating our model's performance:
By carefully reviewing our results and adjusting our model accordingly, we can improve its performance and make more accurate predictions.
Final Thoughts
As you implement and evaluate your Outlier Detection algorithms, it's essential to consider the types of outliers you're dealing with. This will help you choose the right approach for your specific problem.
DBSCAN, as a Density-Based Spatial Clustering of Applications with Noise algorithm, is a popular choice for Outlier Detection. It can be used to identify noise points in your data.
When evaluating your Outlier Detection methods, consider using PyOD as a unified library to streamline your workflow. This library can help you compare different algorithms and choose the best one for your needs.
If you're struggling to identify outliers, you can view Outlier Detection as a Classification problem. This can help you approach the problem from a different angle and get better results.
To summarize, Outlier Detection is a crucial step in Machine Learning projects. By considering the types of outliers and choosing the right algorithm, you can improve the accuracy of your models and get better results.
Advanced Techniques
Outlier detection can be a complex task, but there are many techniques to help you spot those odd bits in your data.
Distribution-based techniques are a great place to start. The Minimum Covariance Determinant and Elliptic Envelope are two popular methods that can help you identify outliers in your data.
Depth-based techniques are another effective way to detect outliers. The Isolation Forest algorithm is a great example of this, and it's often used in combination with other techniques.
Clustering-based techniques can also be useful, particularly the Local Outlier Factor. This method can help you identify outliers by looking at the density of your data.
Density-based techniques are similar to clustering-based techniques, and DBSCAN is a great example of this. This algorithm can help you identify outliers by looking at the density of your data.
There are also unified libraries for outlier detection, such as PyOD, which can make it easier to implement these techniques.
Statistical techniques, like the Interquartile range, can also be used to detect outliers.
Frequently Asked Questions
What is the best algorithm for outlier detection?
There is no single "best" algorithm for outlier detection, as the most effective method depends on the specific data and use case. Popular options include Z-score, IQR, and clustering techniques, which can help data scientists improve model accuracy and reliability.
Sources
- https://builtin.com/data-science/outlier-detection-python
- https://eyer.ai/blog/anomaly-detection-in-time-series-data-python-a-starter-guide/
- https://cnvrg.io/anomaly-detection-python/
- https://datastud.dev/posts/python-outlier-detection/
- https://spotintelligence.com/2023/08/07/outlier-detection-in-machine-learning/
Featured Images: pexels.com