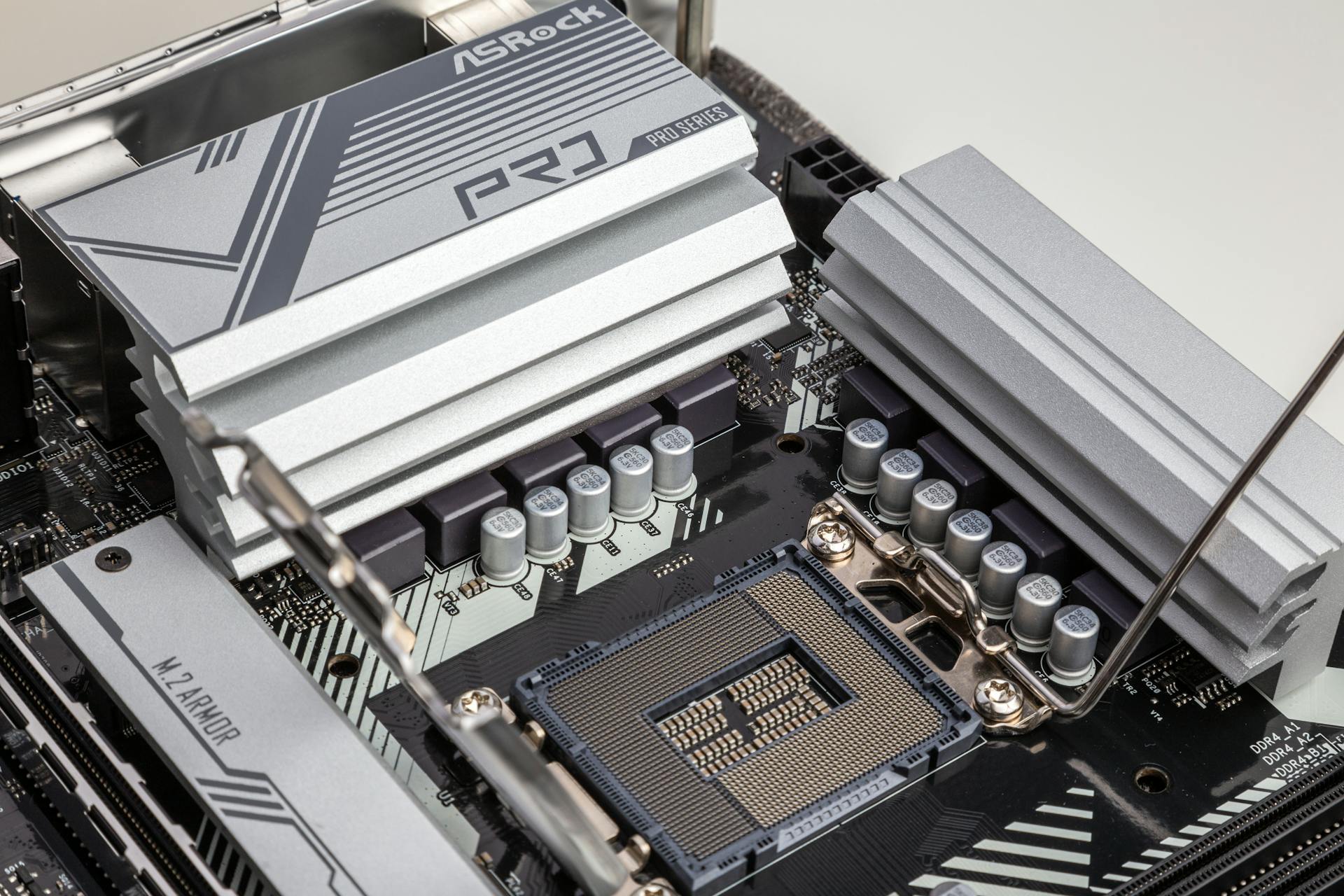
Thread starvation is a critical issue that can significantly impact the performance and reliability of multithreaded applications. It occurs when a thread is unable to access a shared resource, such as a lock or a buffer, for an extended period.
This can happen when a thread is constantly blocked by other threads, preventing it from executing its task. For example, if one thread is holding onto a lock and other threads are waiting to access it, the threads waiting to access the lock will be starved of resources.
Thread starvation can have serious consequences, including increased latency, reduced throughput, and even crashes. It's essential to understand the root causes of thread starvation to prevent it from happening in the first place.
Understanding Thread Starvation
Thread starvation occurs when a thread is unable to make progress because other threads are continuously taking precedence over it in accessing shared resources.
This can happen due to poor resource management, inefficient thread scheduling, or contention for shared resources.
The symptoms of thread starvation can manifest in various ways, including unresponsive or slow performance of a multi-threaded application.
Increased contention for synchronized resources can also occur, leading to further performance issues.
Deadlock situations where threads are waiting indefinitely for access to resources can also arise.
Here are some common signs of thread starvation:
- Unresponsive or slow performance of a multi-threaded application
- Increased contention for synchronized resources
- Deadlock situations where threads are waiting indefinitely for access to resources
Understanding the Checker
The checker is a runtime assertion that measures CPU starvation by looking at latency. It's a simple code that approximates how any other asynchronous event would behave.
The code in question measures not only the CPU but also the overhead associated with IO.sleep. This is a flaw in the test, but it tends to do a good job of isolating the action of enqueuing a new asynchronous event into the Cats Effect runtime and then reacting to that action on the CPU.
The checker is critical because it approximates the way that any other asynchronous event would behave. For example, if a new connection came in on a server socket, the time between when that I/O event is registered by the OS kernel and when the IO.async wrapping around the server socket is fired would converge to delta time.
The default threshold for producing the warning is 100 milliseconds. If you raise the threshold or disable the checker entirely, you are saying that more than 100 milliseconds of latency is acceptable for your application.
Understanding the Issue
Thread starvation is a real issue that can bring even the most well-designed multi-threaded applications to a grinding halt.
Poor resource management is a common culprit behind thread starvation, causing threads to fight for access to shared resources like CPU, memory, or locks.
Inefficient thread scheduling can also contribute to thread starvation, making it difficult for threads to make progress.
Increased contention for synchronized resources is another sign of thread starvation, where multiple threads are constantly competing for access to shared resources.
Here are some common symptoms of thread starvation:
- Unresponsive or slow performance of a multi-threaded application
- Increased contention for synchronized resources
- Deadlock situations where threads are waiting indefinitely for access to resources
Common Causes
Thread starvation can be caused by heavy contention, where multiple threads compete for a limited set of resources, leading to one or more threads being starved of those resources.
Heavy contention can occur when many threads are trying to access the same resource, such as a database or a file. This can cause a bottleneck, where one thread is constantly blocking others from accessing the resource.
Inefficient scheduling is another cause of thread starvation. The JVM's thread scheduler may not allocate CPU time fairly among threads, causing some threads to be starved of processing time.
This can happen when the JVM prioritizes certain threads over others, or when it fails to switch between threads quickly enough.
Unfair locking mechanisms can also lead to thread starvation. This occurs when the use of unfair locking mechanisms, such as the ReentrantLock in Java, continually denies access to certain threads.
Here are some common causes of thread starvation:
Identifying and Troubleshooting
Identifying thread starvation can be challenging, but there are several indicators to look out for, such as high CPU usage with low throughput or thread contention or deadlock warnings in application logs.
Thread dumps can provide valuable insights into the state of threads within the application. Tools like jstack or visualVM can be used to capture and analyze thread dumps, helping to identify threads that are blocked or waiting for resources.
Unresponsive behavior or slow performance under concurrent load can also be a sign of thread starvation. This is because threads are competing for resources, leading to a bottleneck in the application.
Analyzing thread dumps can help pinpoint potential areas of contention or resource starvation. By examining thread states and stack traces, you can identify threads that are waiting for resources.
Here are some common signs of thread starvation:
- High CPU usage with low throughput
- Thread contention or deadlock warnings in application logs
- Unresponsive behavior or slow performance under concurrent load
Using a Java profiler can help identify hotspots in the code where threads are contending for resources. Profiling the application under load can reveal inefficient synchronization, long-running operations, or bottlenecked resources that contribute to thread starvation.
Optimizing Synchronization and Resource Pooling
Optimizing Synchronization and Resource Pooling is crucial to preventing thread starvation. Implementing resource pooling strategies like connection pooling for database access or object pooling for expensive resources can alleviate contention and reduce thread starvation.
Efficiently managing shared resources ensures fair access for all threads. By using finer-grained locks, employing non-blocking algorithms, or re-evaluating the use of synchronized blocks, you can reduce contention and minimize the risk of thread starvation.
Reviewing the use of locks, synchronized blocks, and concurrent data structures in your code can uncover opportunities for improving thread synchronization. This can be done by using async profilers to analyze thread behavior.
In heavily compute-bound applications, maintaining fairness is key. The solution often involves a three-step process: increasing the granularity of computation, adding IO.cedes around computationally-intensive functions, and restricting outstanding compute-heavy work to a fraction of the total number of physical threads.
Here's a summary of the three steps:
- Try to increase the granularity of your computation by splitting it into multiple IO steps
- Add IO.cedes around your computationally-intensive functions
- Restrict the amount of outstanding compute-heavy work to some fraction of the total number of physical threads (half is a good starting point)
Sources
- https://javanexus.com/blog/troubleshooting-java-concurrency-starvation
- https://typelevel.org/cats-effect/docs/core/starvation-and-tuning
- https://www.geeksforgeeks.org/deadlock-starvation-and-livelock/
- https://en.wikipedia.org/wiki/Starvation_(computer_science)
- https://www.linkedin.com/pulse/starvation-multithreading-understanding-causes-pavan-pothuganti-faknc
Featured Images: pexels.com